Beliefs¶
A belief is specified by the base space \(X\) as well as the chosen set of sufficient statistics \(\phi(x)\). Any variable type defines an exponential family distribution
indexed by the natural parameter \(\lambda\). We will denote by \(b \in \lambda\) the natural parameter associated to the statistic \(x \in \phi(x)\). The log-partition, mean and variance are then given by:
The second moment is given by \(\tau(\lambda) = r(\lambda)^2 + v(\lambda)\)
In Tree-AMP, a belief is implemented as a python submodule of tramp.beliefs
containing the functions A()
,
r()
, v()
, tau()
and some additional functions if relevant.
As a sanity check, you can use the tramp.checks.plot_belief_grad_b()
that will check that \(r = \partial_b A\) and \(v = \partial_b^2 A\).
See the various beliefs below for concrete examples.
If you implement a new belief, please make sure that it passes the gradient
checking and add it to the test suite
tramp.tests.test_beliefs.test_belief_grad_b()
.
Binary¶
The log-partition, mean and variance are given by
The corresponding exponential family distribution is the Bernoulli
where the natural parameter \(b = \tfrac{1}{2}\ln\tfrac{p_+}{p_-}\) is (half) the log-odds.
>>> from tramp.beliefs import binary
>>> from tramp.checks import plot_belief_grad_b
>>> plot_belief_grad_b(binary)
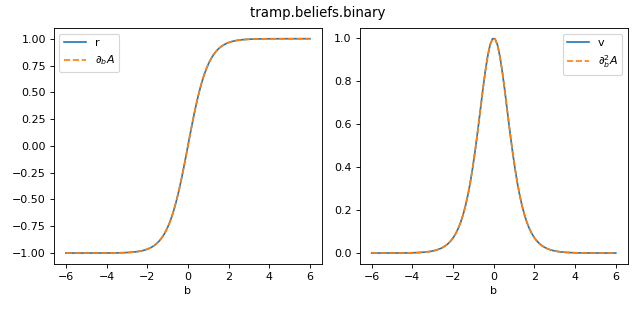
Normal¶
The log-partition, mean and variance are given by
The corresponding exponential family distribution is the Normal
of mean \(r=\frac{b}{a}\) and variance \(v=\frac{1}{a}\)
>>> from tramp.beliefs import normal
>>> from tramp.checks import plot_belief_grad_b
>>> plot_belief_grad_b(normal, a=1)
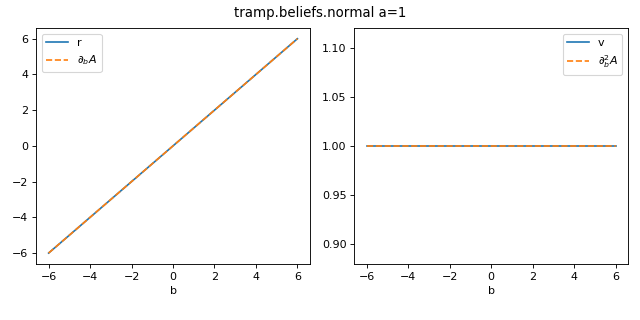
Sparse¶
The log-partition, mean and variance are given by
where \(A(a,b), r=r(a,b), v=v(a,b)\) are the log-partition, mean and variance of a Normal variable, \(\sigma(\xi)\) is the sigmoid and \(\xi = A(a,b) - \eta\).
Besides there is a finite probability \(p(x=0 | a,b,\eta) = 1 - \sigma(\xi)\) that \(x\) is exactly zero. We will denote its complementary by
The corresponding exponential family distribution is the Gauss-Bernoulli
with fraction of non-zero elements \(\rho = p(a,b,\eta)\)
>>> from tramp.beliefs import sparse
>>> from tramp.checks import plot_belief_grad_b
>>> plot_belief_grad_b(sparse, a=1, eta=2)
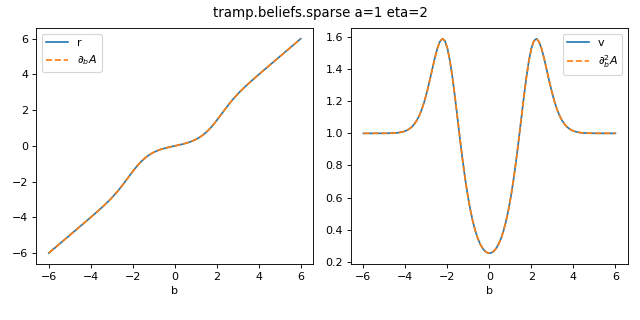
Mixture¶
We consider a K-mixture Normal variable with natural parameters \(a = \{a_k\}_{k=1}^K\), \(b = \{b_k\}_{k=1}^K\) and \(\eta = \{\eta_k\}_{k=1}^K\). The log-partition, mean and variance are given by
where \(A(a_k, b_k), r_k = r(a_k, b_k), v_k = v(a_k, b_k)\) are the log-parition, mean and variance of the \(k^{th}\) Normal variable, \(\sigma = \mathrm{softmax}(\xi)\) and \(\xi_k = \eta_k + A(a_k, b_k)\).
Besides the probability to belong to each of the K-components is
The corresponding exponential family distribution is the Gaussian mixture
On the plot below, the gradients of \(A(a,b+b_0,\eta)\) are taken with respect to scalar \(b\) for fixed 2-components \(a, b_0, \eta\) and are checked against the corresponding \(r(a,b+b_0,\eta)\) and \(v(a,b+b_0,\eta)\).
>>> from tramp.beliefs import mixture
>>> from tramp.checks import plot_belief_grad_b
>>> plot_belief_grad_b(mixture, a=np.ones(2), b0=np.array([-1, +1]), eta=np.ones(2))
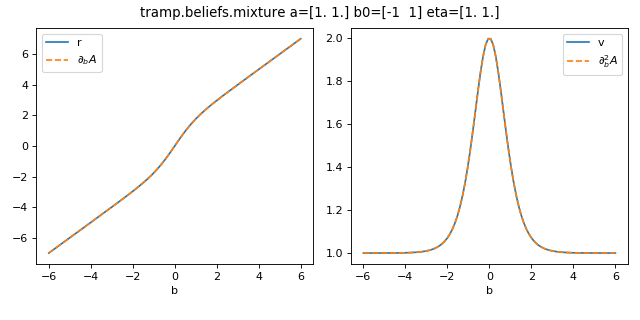
Truncated¶
We consider a truncated Normal variable restricted to the interval \(X = [x_\min, x_\max]\). The log-partition, mean and variance are given by
where \(A(a,b), r=r(a,b), v=v(a,b)\) are the log-partition, mean and variance of a Normal variable.
\(p_Z\) denotes the probabilty that the standard Normal \(z \sim \mathcal{N}(0,1)\) falls withing the rescaled interval \(Z = \frac{X - r}{\sqrt{v}} = [z_\min, z_\max]\)
with \(\Phi\) the Normal cumulative distribution function.
It is equal to the probabilty \(p_X(r, v)\) that the Normal \(x \sim \mathcal{N}(r, v)\) falls within the \(X\) interval:
\(r_Z\) denotes the mean and \(v_Z\) the variance of the standard Normal \(z \sim \mathcal{N}(0,1)\) restricted to the \(Z\) interval:
Warning
Computing \(r_Z\), \(v_Z\) and \(\ln p_Z\) is numerically tricky, especially in the tails. We follow the implementation suggested in the TruncatedNormal.jl Julia package.
The corresponding exponential family distribution is the truncated Normal
>>> from tramp.beliefs import truncated
>>> from tramp.checks import plot_belief_grad_b
>>> plot_belief_grad_b(truncated, a=1, xmin=-1, xmax=1)
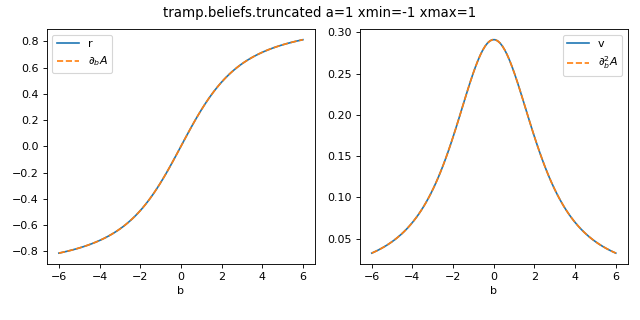
Positive¶
It corresponds to a Normal variable resticted to \(X=\mathbb{R}_+\) and a special case of the Truncated variable with \(x_\min=0\) and \(x_\max = +\infty\).
>>> from tramp.beliefs import positive
>>> from tramp.checks import plot_belief_grad_b
>>> plot_belief_grad_b(positive, a=1)
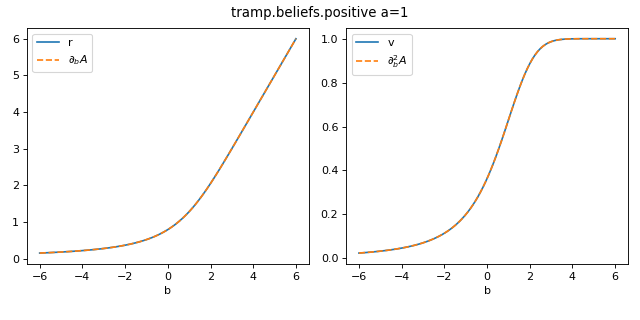
Exponential¶
The log-partition, mean and variance are given by
The corresponding exponential family distribution is the Exponential with mean \(r = -\frac{1}{b}\)
The Exponential variable is also the limit \(a\rightarrow 0\) of the Positive variable:
Note
The natural parameter \(b\) must be negative.
>>> from tramp.beliefs import exponential
>>> from tramp.checks import plot_belief_grad_b
>>> plot_belief_grad_b(exponential)
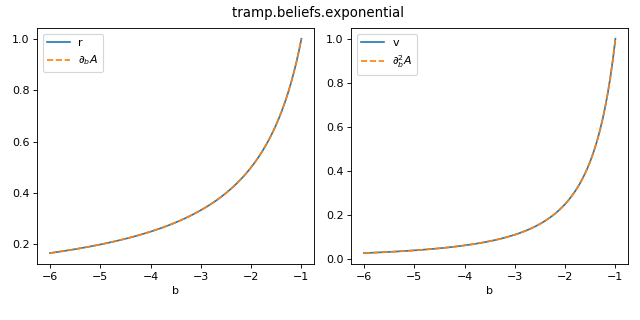